If you created a React project using Create React App or a custom project template, you might have noticed that it uses Webpack and Babel. But what do they do?
In this post, I will explain what each of these tools are and their purpose in a React project.
Webpack
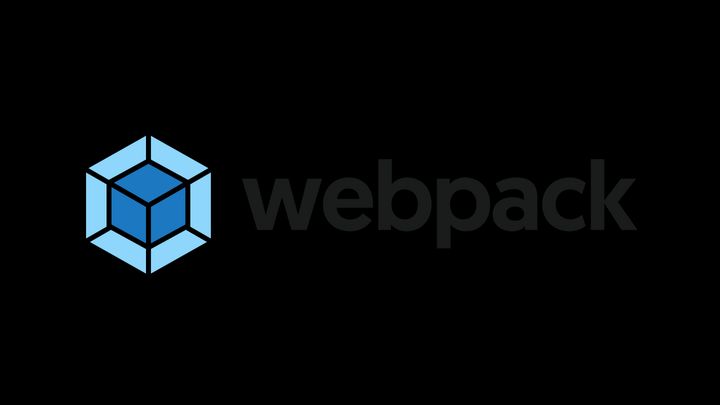
What is Webpack?
Webpack is an open source module bundler. A module bundler is a program that combines separate code modules into a single "bundle" that is more efficient to distribute.
Javascript did not support modules originally. It did not have the ability to import or export code across different files. Webpack was originally developed in that era. Support for modules was being added to Javascript in the ECMAScript 2015 specification of the language (also known as ES6) but many browsers did not support it yet. Webpack had support for it and this allowed us to use modules during development by using Webpack to "bundle" these modules together into a single script that could run on the browser.
Eventually browsers gained native support for modules. But it was still not efficient to load the thousands of modules that a large web app consists of using separate network requests. So we still bundle web apps before they are published.
What is Webpack used for in a React project?
In a React project, Webpack is used to handle all of the import statements. It starts at a single file which is called an entrypoint and then recursively goes through each imported file. It then generates a single "bundled" file that contains all of the imported files.
In most React projects, Webpack does more than just combining separate Javascript modules. Webpack can be setup to run different "loaders" for different file types and we can use this to have Webpack process other types of imported files are well. For example, we can setup webpack to process imported CSS files and to also bundle them into a single CSS file.
So in summary, Webpack is used to process all of the separate code and asset files in a React project to create "bundles" that can be efficiently distributed.
Babel
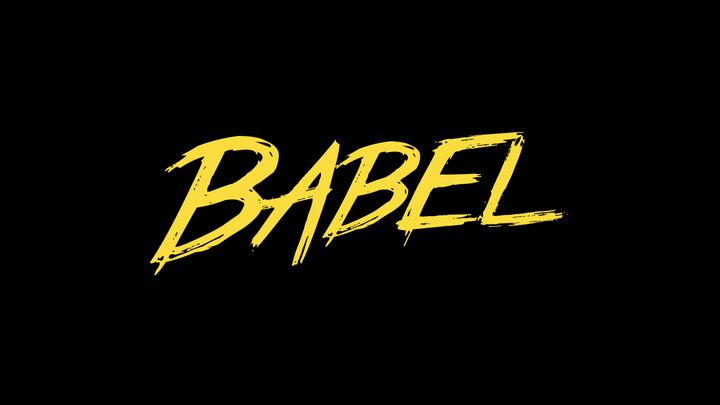
What is Babel?
Babel is an open source transpiler. It is used to convert different types of Javascript syntax and syntax extensions into code that browsers can run. Let's go over what this means.
Javascript has changed a lot since it was first created. The language itself is specified in a specification called the ECMAScript Language Specification and Javascript engine follow that specification. (A Javascript engine is the component, either in a web browser or stand alone, that executes Javascript code)
New features are added to the ECMAScript spec on a regular basis. But it usually takes some time for the different engines to start supporting these new features. This is where Babel comes in.
Babel can convert JS code between different versions of the ECMAScript spec. This means we can use features from the latest edition of the ECMAScript spec, "transpile" it with Babel and get code in a lower version of the ECMAScript spec that a browser can run.
Babel is also used to tackle Javascript syntax extensions. These are languages or more accurately "syntaxes" that build on top of the ECMAScript spec to add even more features. (e.g. JSX, Typescript) The code that is written using these syntaxes can't run directly on an engine since they use features that are not in the ECMAScript spec. But we can use Babel to also transpile this code into runnable code.
What is Babel used for in a React project?
React uses a Javascript syntax extension called JSX for specifying the HTML markup of a React component. In the example below, the value that is returned by the Greeting component uses JSX.
const Greeting = () => {
const name = 'bash';
return (
<h1>Hey {name}!</h1>
)
}
Since JSX is a Javascript syntax extension it can't run directly in the browser. This is where we need Babel to transpile the JSX code into code that a browser can run. Most React projects have Webpack setup to pass the code written in .js
or .jsx
files through Babel before it is bundled together.
Moreover, we are also able to start using new features in the ECMAScript spec before they are widely supported thanks to Babel. It can transpile the unsupported features into equivalent code that can work on browsers in the wild.
Next steps
Now that you know the purpose of Webpack and Babel in a React project, you can see how it works by setting it up yourself using this guide.